First the <script> Tag, Then Controversy
At first, HTML limited itself to text oriented elements, which got processed in a very static manner. Mosaic, one of the most popular of the early browsers, wouldn’t display anything until all HTML had finished downloading. On an early ‘90s dial up connection, this could leave a user staring at a blank screen for literally minutes. Netscape Navigator exploded in popularity almost as soon as it appeared in the mid to late ‘90s. Like a lot of current disruptive innovators, Netscape pushed boundaries with changes that weren’t universally liked . One of Navigator’s many innovations was rendering HTML as it downloaded, allowing users to begin reading a page as soon as possible, signaling the end for Mosaic in the process. In a famous 10 day period in 1995, Brendan Eich created JavaScript for Netscape. They didn’t originate the idea of dynamically scripting a web page – ViolaWWW preceded them by 5 years – but much like Isaac Singer’s sewing machine, their popularity made them synonymous with the concept. The implementation of the <script> tag went back to blocking HTML download and rendering. The limited communication resources commonly available at the time couldn’t handle fetching two data sources simultaneously, so when the browser saw <script> in the markup, it would pause HTML execution and switch to handling JS. In addition, any JS actions that affected HTML rendering, done via the browser-supplied API called DOM, placed a computational strain on even that day’s cutting edge Pentium CPUs. So when the JavaScript had finished downloading, it would parse and execute, and only after that pick up processing HTML where it had left off. At first, very few coders did any substantial JS work. Even the name suggested that JavaScript was a lesser citizen compared to its server-side relatives like Java and ASP. Most JavaScript around the turn of the century limited itself to client side conditions the server couldn’t affect – often simple form activities like putting focus into the first field, or validating form input prior to submitting. The most common meaning of AJAX still referred to the caustic household cleaner, and almost all nontrivial actions required the full HTTP round trip to the server and back, so almost all web developers were backenders who looked down on the “toy” language. Did you catch the gotcha in the last paragraph? Validating one form input might be simple, but validating multiple inputs on multiple forms gets complicated – and sure enough, so did JS codebases. Just as fast as it became apparent that client side scripting had undeniable usability benefits, so too the problems with vanilla script tags emerged: unpredictability with notification of DOM readiness; variable collisions in file concatenation; dependency management; you name it. JS developers had a very easy time finding jobs, and a very hard time enjoying them. When jQuery appeared in 2006, developers adopted it warmly. Today, 65 to 70% of the top 10 million websites have jQuery installed. But it never intended and could offer little to solve the architectural issues: the “toy” language had made it to the big time, and needed big time skills.What Exactly Did We Need?
Fortunately, other languages had already hit this complexity barrier, and found a solution: modular programming. Modules encouraged lots of best practices:- Separation: Code needs to be separated into smaller chunks in order to be understandable. Best practices recommend these chunks should take the form of files.
- Composability: You want code in one file but reused in many others. This promotes flexibility in a codebase.
- Dependency management: 65% of sites might have jQuery installed, but what if your product is a site add-on, and needs a specific version? You want to reuse the installed version if it’s suitable, and load it if it’s not.
- Namespace management: Similar to dependency management – can you move the location of a file without rewriting core code?
- Consistent implementation: Everybody should not come up with their own solution to the same problems.
Early solutions
Each solution to these problems which JavaScript developers came up with had an influence on the structure of ES6 modules. We’ll review the major milestones in their evolution, and what the community learned at each step, finally showing the results in the form of today’s ES6 modules.- Object Literal pattern
- IIFE/Revealing Module pattern
- CommonJS
- AMD
- UMD
Object Literal pattern
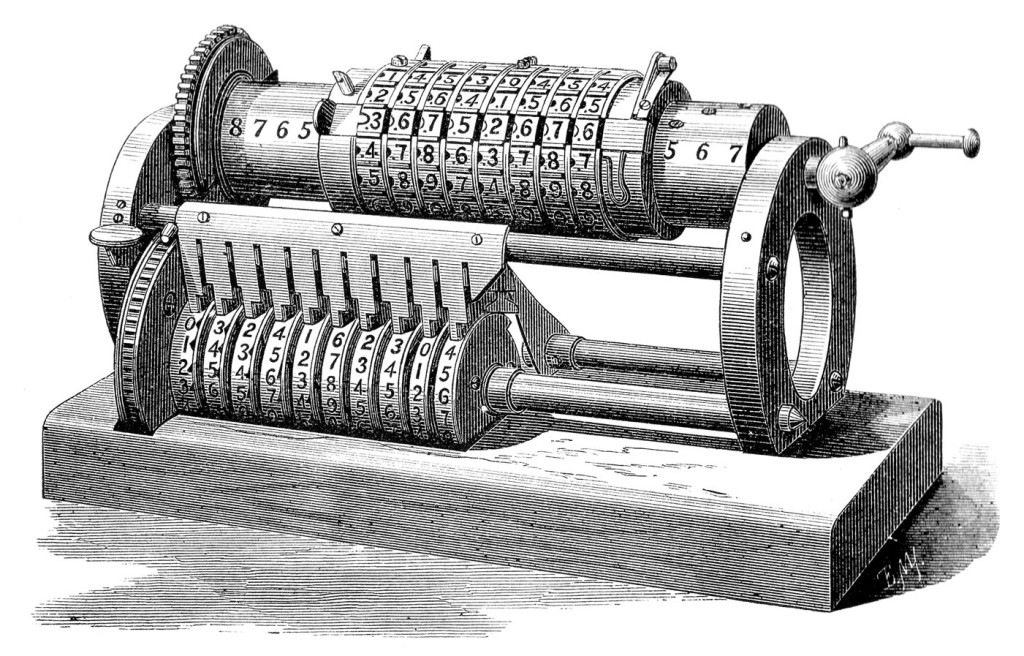
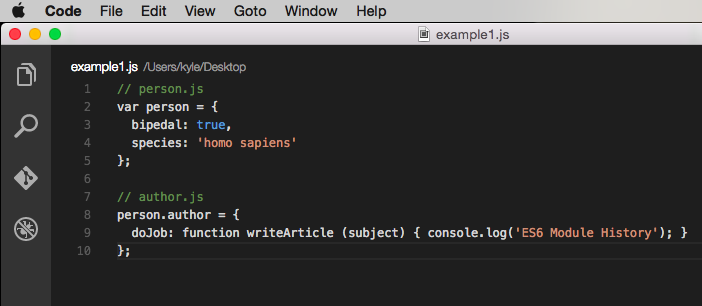
<!DOCTYPE html>
<html>
<head>
<script src="person.js"></script>
<script src="author.js"></script>
</head>
<body>
<script>
person.author.doJob('ES6 module history');
</script>
</body>
<script>
// shared scope means other code can inadvertently destroy ours
var person ='all gone!';
</script>
</html>
What it offered
This approach’s primary benefit was ease in understanding and implementation. Many other aspects were not at all easy.What held it back
It relied on a variable in the global scope (person) as its root; if some other JS on the page declared a variable by the same name, your code would disappear without a trace! In addition, there’s no reusability – if we wanted to support a monkey banging on a typewriter, we’d have to duplicate the author.js file: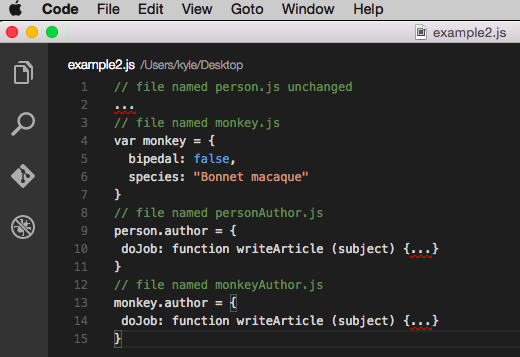
IIFE/Revealing Module pattern
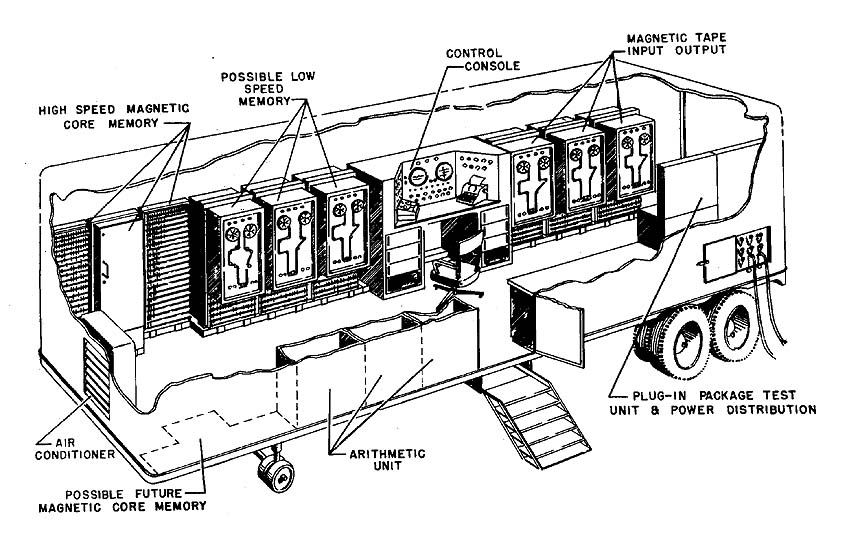
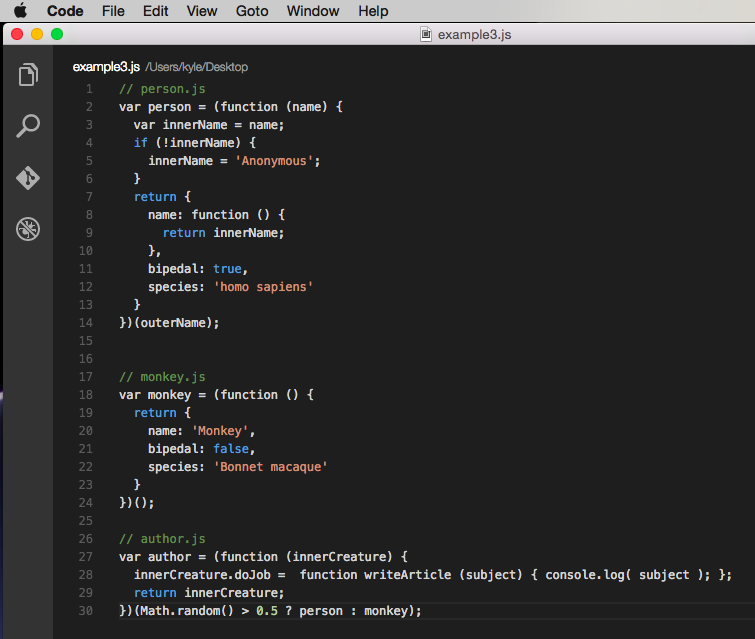
// IIFEPage.html
<!DOCTYPE html>
<html>
<head>
<script>
// set init values
var outerName ='Ross';
</script>
<script src="person.js"></script>
<script src="monkey.js"></script>
<script src="author.js"></script>
<script>
// we can get rid of person after author has loaded....
person =undefined;
</script>
</head>
<body>
<script>
// ...and author will still work here
author.doJob('ES6 Module History');
</script>
</body>
</html>
What it offered
Due to closure, we have a lot of control inside the IIFE. For example,innerName
is essentially private, because nothing outside can access it – we choose to reveal access to it through the object property called name. We have constructor-like functionality, like setting a default value for name inside person. We have control of importing dependencies – if we need to rename something from the outside world (often jQuery’s “$” alias), we can take the exterior name in the arguments and give it any arbitrary name in the interior. Finally, combining these techniques allows us to implement the decorator pattern in author, which begins to decouple that code from its dependence on the creature which is passed to it.
What held it back
The IIFE/Revealing Module offered many features, and as we’ll see, had a strong influence on AMD. But the syntax was ugly, it was an ad-hoc hack without a standard, and it still relies heavily on global scope.CommonJS
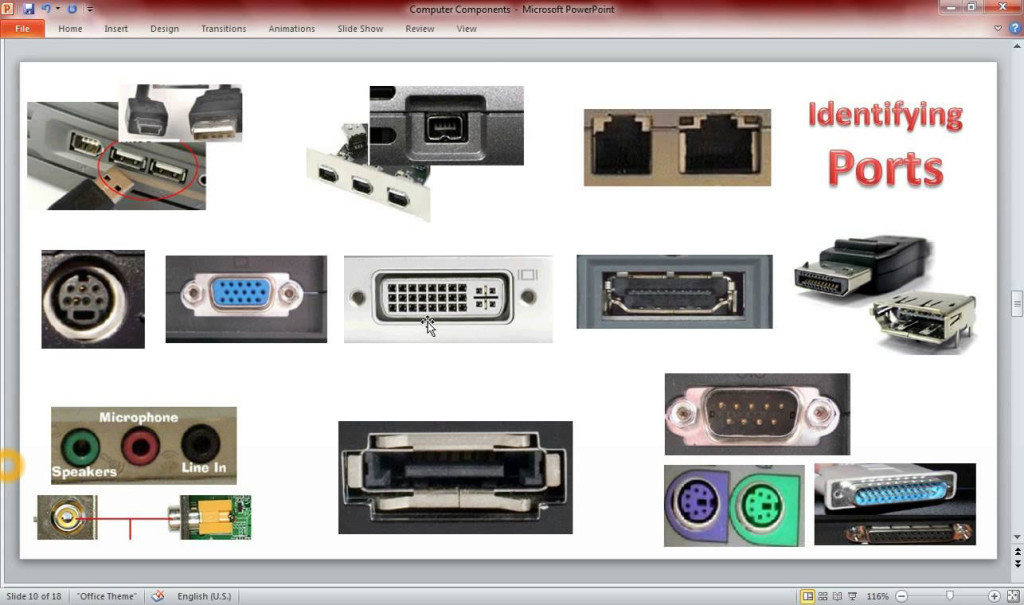
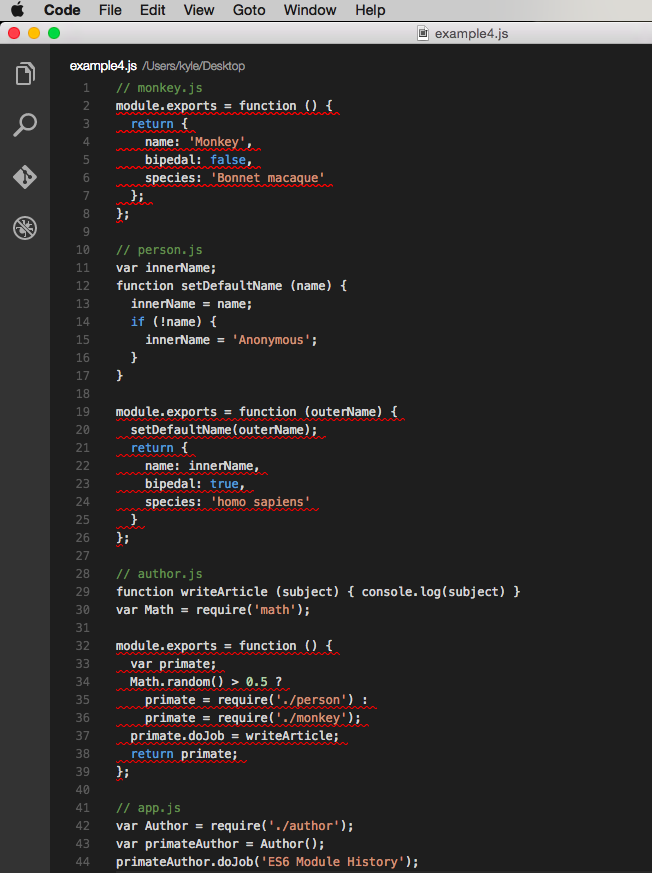
// commonJSPage.html
<!DOCTYPE html>
<html>
<head>
<script src="out/bundle.js"></script>
</head>
<body>
<script>
</script>
</body>
</html>
What it offered
Given that external requirement for a loader, the syntax is clear and concise, and directly influenced ES6 module syntax. Also, modules limit variable scope to within themselves; it’s no longer even possible to declare a global.What held it back
As a downside, CJS doesn’t play well in an asynchronous environment. All require calls have to be executed before code can proceed. This explains the pre-compilation step earlier, but it also makes “lazy loading” really difficult – all code needs to be loaded before any execution can begin. Not awesome on slow connections or underpowered devices.AMD
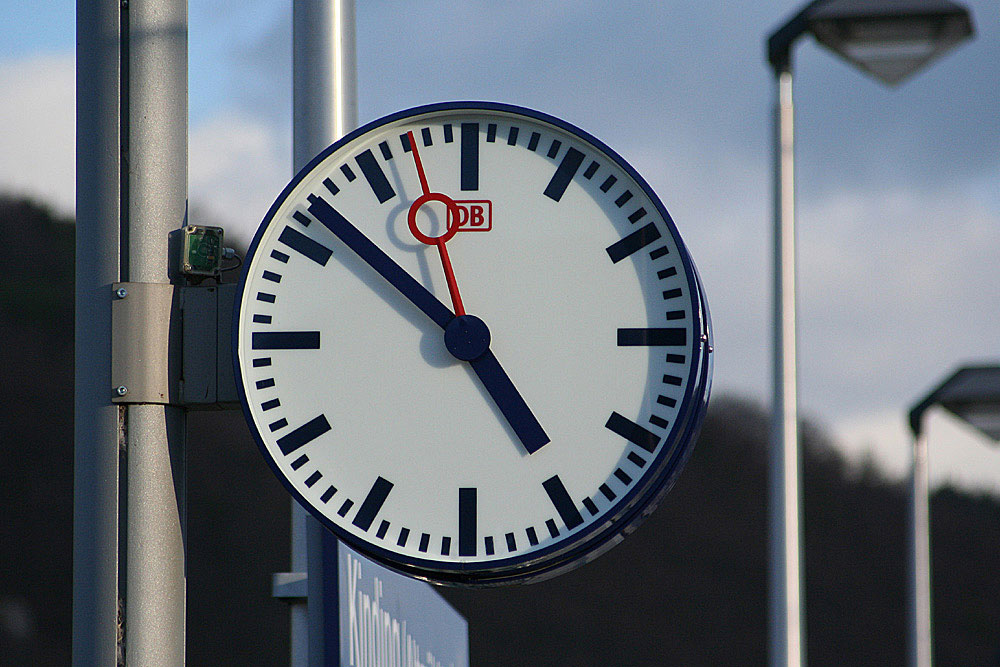
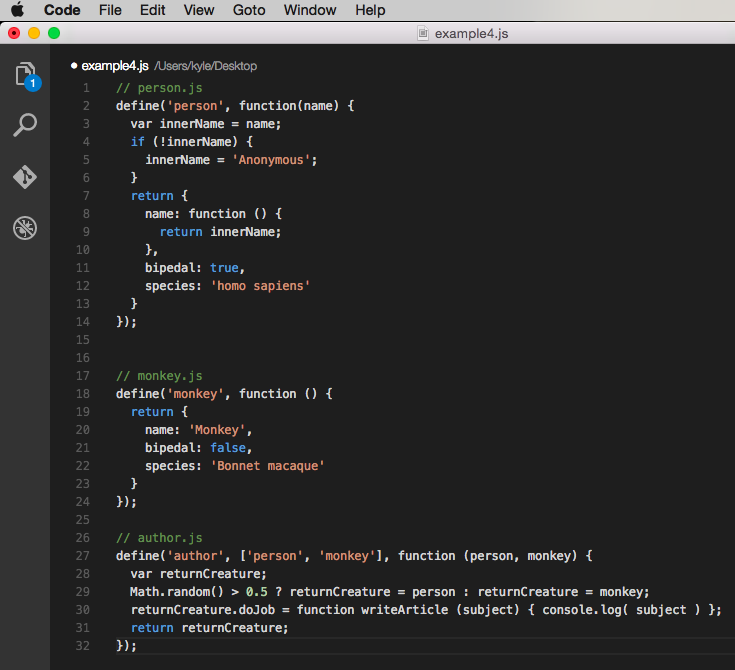
// AMDPage.html
<!DOCTYPE html>
<html>
<head>
<script src="script/require.js"></script>
</head>
<body>
<script>
require.config({
baseUrl:"script",
paths: {
"person":"person"
}
});
require(["author"],function(author) {
author.doJob('ES6 Module History');
});
</script>
</body>
</html>
Pros and cons
AMD ruled hard for many years, but leaned heavily on an ugly syntax which required a lot of boilerplate punctuation. Its asynchronous nature, which the browser demanded, meant it couldn’t be statically analyzed, and there were other similar small reasons that it wasn’t the solution for everyone.UMD
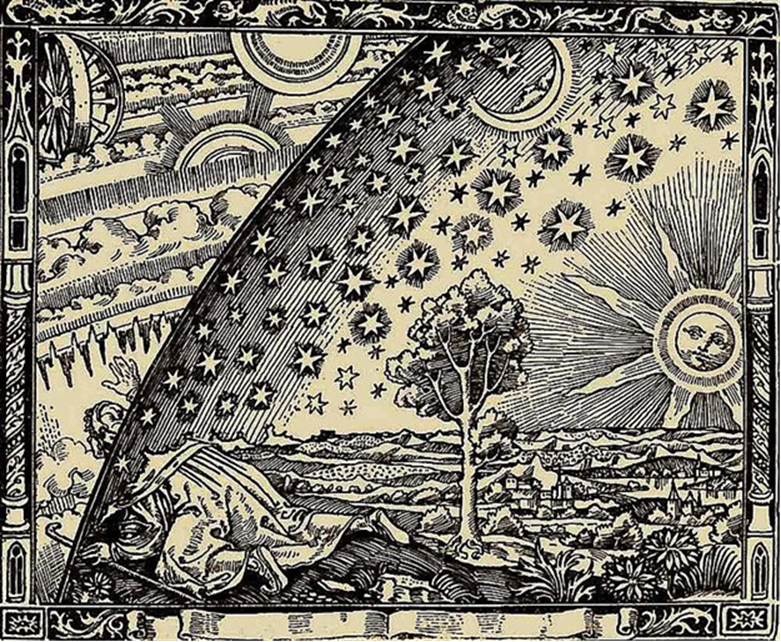
Pros and cons
Like most attempts at having cake and also eating it, it suffered from the drawbacks of both parents without providing a lot of benefits. Mentioned here for historical completeness, it did take the first step towards the holy grail of Isomorphic JavaScript, which can run on both the server and client.ES6 modules
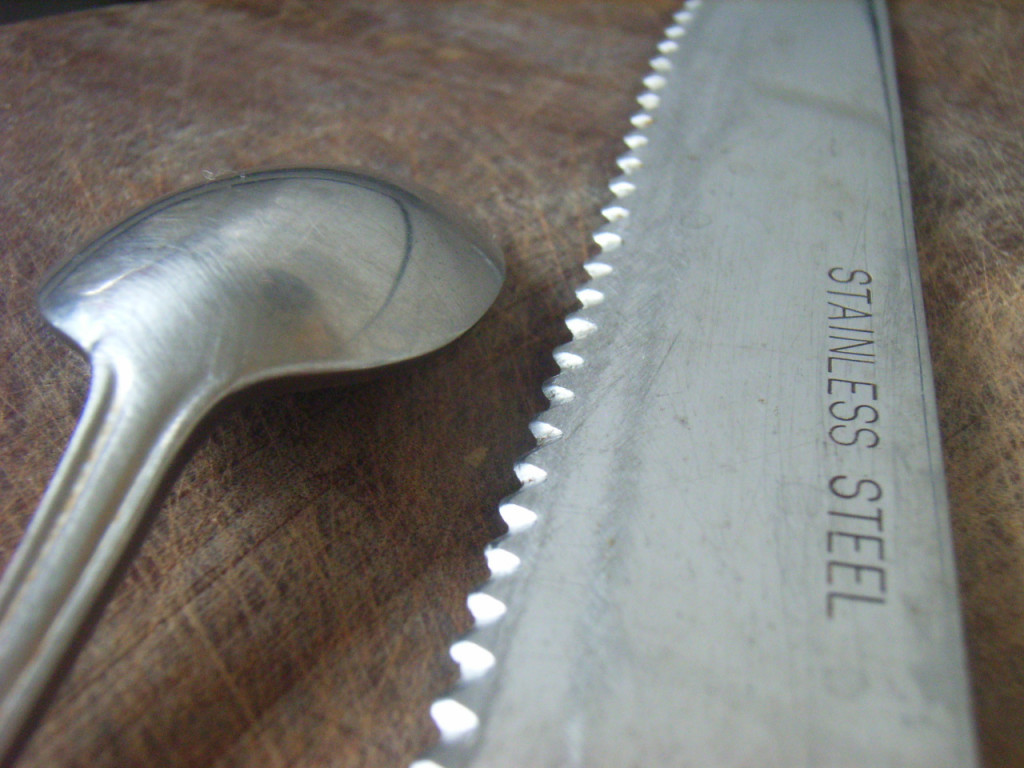
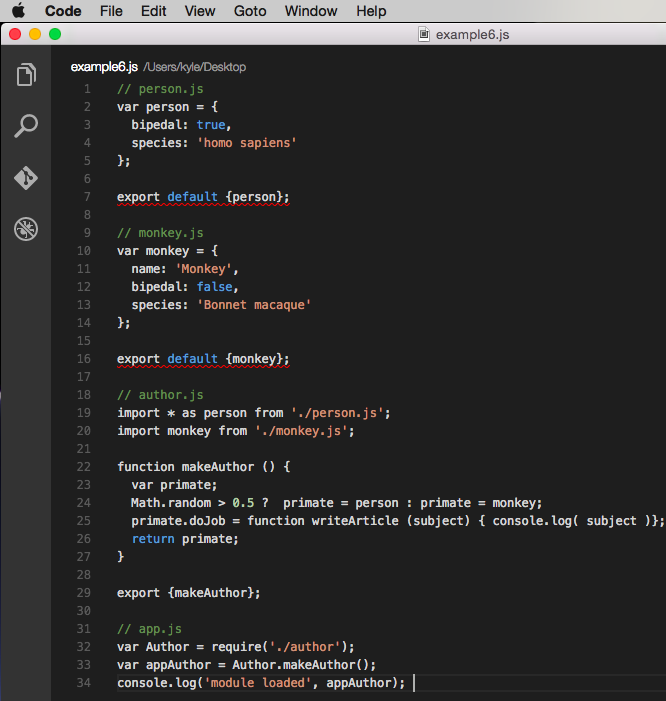
<!DOCTYPE html>
<html>
<head>
<script src="./out/bundle.js"></script>
</head>
<body>
<script>
console.log('page loaded');
</script>
</body>
</html>
Only one small problem remains: the front end ecosystem barely supports ES6 modules. No browser natively supports the new import and export keywords, or the proposed HTML5 module element. Tools called transpilers, like Babel and Traceur, can precompile ES6 modules into valid ES5 code, which today’s browsers can process; but that ES5 has to be wrapped in an asynchronous syntax and then handled by a script loader like RequireJS, Browserify or SystemJS.
Trying to pass even a trivial ES6 module through these two abstract layers, transpilation and asynchronous loading, tends to create implementation challenges. While putting together this example, I had a run-time (browser) error that a require statement couldn’t find its dependency. I knew Browserify sometimes needs the dot-slash directory prefix to identify modules (‘./author’), but that broke my Babel build, as I had it setup to run a directory above the Browserify bundle. I’ve had similar issues with Babel and Webpack in a production app.
Point is, this is bleeding edge stuff. It’s not impossible to implement, given time, but expect to spend more time on configuration and troubleshooting than with a more established technique, like AMD + RequireJS.
Conclusion
The history of JavaScript module techniques mirrors the explosion and evolution of the internet itself. Just as mainframes no longer controlled helpless terminals, centralized control organizations like standards committees could no longer issue orders and expect the multitudes to silently obey. Individual contributors devised their own solutions to the problems they thought most important, and popular adoption represented votes for those solutions. After different implementations have explored permutations of an idea, standards follow, to capture the learning. This attitude – pithily summed up by Steve Jobs as “real artists ship” – embody the egalitarian and pragmatic attitude that makes modern web development so successful and exciting. This article is part of the web development series from Microsoft tech evangelists and DevelopIntelligence on practical JavaScript learning, open source projects, and interoperability best practices including Microsoft Edge browser and the new EdgeHTML rendering engine. DevelopIntelligence offers instructor-led JavaScript Training and React Training for technical teams and organizations. We encourage you to test across browsers and devices including Microsoft Edge – the default browser for Windows 10 – with free tools on dev.microsoftedge.com, including F12 developer tools — seven distinct, fully-documented tools to help you debug, test, and speed up your webpages. Also, visit the Edge blog to stay updated and informed from Microsoft developers and experts.Frequently Asked Questions (FAQs) about ES6 Modules
What is the main difference between ES6 modules and CommonJS modules?
ES6 modules and CommonJS modules are both popular systems for managing JavaScript modules, but they have some key differences. The most significant difference is that ES6 modules use a static module structure, meaning the module structure is determined at compile time, not runtime. This allows for better static analysis, including tree shaking for eliminating unused exports and predicting what will be imported. On the other hand, CommonJS modules are dynamic, meaning they are loaded and interpreted at runtime, which can be more flexible but less efficient.
How can I use ES6 modules in Node.js?
Node.js has traditionally used the CommonJS module system, but it has added support for ES6 modules in more recent versions. To use ES6 modules in Node.js, you need to use the .mjs file extension or set “type”: “module” in your package.json file. Then, you can use import and export syntax as you would in a browser environment.
Can I use ES6 modules in older browsers?
Older browsers do not natively support ES6 modules. However, you can use tools like Babel and Webpack to transpile your code into a format that older browsers can understand. These tools will convert your ES6 module syntax into equivalent CommonJS or AMD syntax that is compatible with older browsers.
What is the benefit of using ES6 modules over global variables?
Using ES6 modules provides several benefits over using global variables. First, modules help avoid naming conflicts by keeping variables and functions scoped to the module they are defined in. Second, modules make it easier to organize and manage your code by breaking it up into smaller, self-contained pieces. Finally, modules can improve performance by allowing for lazy loading and more efficient bundling of code.
How can I import a default export from an ES6 module?
To import a default export from an ES6 module, you can use the import statement followed by the name you want to assign to the default export and the path to the module. For example, import myDefault from './myModule';
will import the default export from myModule.js and assign it to the variable myDefault.
Can I import multiple exports from an ES6 module?
Yes, you can import multiple exports from an ES6 module using the import statement. To import named exports, you can list them in curly braces after the import keyword. For example, import {export1, export2} from './myModule';
will import the named exports export1 and export2 from myModule.js.
Can I use ES6 modules with React?
Yes, you can use ES6 modules with React. In fact, using ES6 modules is the recommended way to import and export components in React. This allows you to take advantage of the benefits of modules, such as better code organization and more efficient bundling.
What is tree shaking and how does it relate to ES6 modules?
Tree shaking is a technique used in modern JavaScript bundlers like Webpack and Rollup to eliminate unused code from the final bundle. Because ES6 modules have a static structure, bundlers can analyze the imports and exports at compile time and exclude any exports that are not used. This can significantly reduce the size of the final bundle and improve performance.
Can I mix ES6 modules and CommonJS modules in the same project?
While it is technically possible to mix ES6 modules and CommonJS modules in the same project, it is generally not recommended. Mixing module systems can lead to confusion and unexpected behavior. If you need to use a CommonJS module in a project that uses ES6 modules, consider using a tool like Babel to transpile the CommonJS module to ES6 syntax.
How can I export multiple values from an ES6 module?
To export multiple values from an ES6 module, you can use the export keyword followed by the values you want to export. You can export any number of values, and they can be any type of JavaScript value, including functions, objects, and primitive values. For example, export {value1, value2, value3};
will export the values value1, value2, and value3 from the module.

Elias Carlston consults for DevelopIntelligence and has over 15 years experience as a software engineer. He has worked for Athenahealth, Zipcar and has been freelancing since 2011. A lifelong rock'n'roll musician, Elias began his entrepreneurial experience as a guitar teacher. He lives in Austin with his wife Amy and two goofy dogs.